Database schemas can be altered by transferring data, like adding or removing tables and adjusting the types of constraints. When you’re making changes to the requirements for an application for a collaborative project, you may want to look into changing the database. In general, updating the .sql data file can be shared; however, this isn’t suitable nor doable, as mistakes like not importing or importing an old version can occur.
Migrations function as the database’s version control, which allows your team members to share and define the schema for your application’s database. Laravel migrations are crucial because they allow you to tackle problems with database collaboration as the entire team. The team members don’t have to update their databases manually each time they pull your changes off source control.
This blog will look at how to build, run and control Laravel migrations.
What are Laravel Migrations?
Laravel Migration is an instruction set that defines the changes you’d like to make to your database schema. The changes could involve:
- Creating new tables.
- Changing the existing ones.
- Adding and altering columns.
- Seeding the database with data that was initially.
In encapsulating the changes into the migration files, Laravel assures the schema of your databases stays in sync with your application’s codebase. This makes monitoring changes to the database across multiple settings for development and deployment much easier.
Laravel lets you perform migrations without having to worry about the particular databases you’re running, regardless of whether the system is MySQL, PostgreSQL, SQLite, or any other that Laravel supports. It abstracts the specific syntax of the database, making migrations adaptable and portable to various database systems.
How to Conduct Laravel Migrations?
Laravel migrations offer a well-organized way of managing schema changes in your database. They can ensure a smooth and consistent change in your application’s database structure. If you’re not familiar with this procedure, don’t hesitate to ask for help from our laravel development team. We’re always here to assist.
Create a Migration File
The first step in preparing your Laravel migration is to create the migration file. The file is the template for the changes you must make to your database schema.
Command: Open your terminal, then navigate the Laravel directories for your project. Follow the steps below:
php artisan make:migration
Replace: Replace the placeholder with a descriptive title that clearly expresses the goal for your move. For example, if your migration creates a “users” table, name it “create_users_table”.
Understanding the Generated File
Location: Artisan will create a new migration file in your project’s migrations and databases directory.
Content: The file will contain a PHP class extending the Illuminate/Database/Migrations/Migration class. It will also include two essential methods: up() and down().
This new file serves as a clean surface to define the precise modifications you intend to bring to your database schema. Once you have the base laid the foundation, you can proceed to the next stage – which is defining the schema.
Define the Schema
After establishing the basis of a new file for migration, we can now determine the schema, or structure, for your data. This is the process of creating the structure of your database by providing each room (tables) and their functionality (columns).
Import the Schema in your migration files; near the very beginning, include an additional line that imports the needed tools:
use Illuminate\Support\Facades\Schema
Employ the up Method
Within the process you created in the migration class, use the potential of Schema Builder in Laravel. To establish your columns and tables, make use of methods such as:
$table($tableName table($tableName, function ($table) ). This technique lets you create the table you want to create. It gives its name and gives closure to the structure.
$table->bigIncrements(‘id’). The method generates a key column “id” with an auto-incrementing Big Integer data type.
$table->string(‘name’, 255). This method generates a name column with the string data type with a maximum size of 255 characters.
public function is up()
{
Schema::create(‘users’, function (Blueprint $table) {
$table->id();
$table->string(‘name’);
$table->string(’email’)->unique();
$table->timestamp(’email_verified_at’)->nullable();
$table->string(‘password’);
$table->rememberToken();
$table->timestamps();
});
}
Follow these steps and use the features of the Schema Builder in Laravel. This will assist you in defining the ideal structure for your database during the migration, allowing you to start making those changes during your next stage.
Use the Method to Implement the Following() Method
The up() method is a crucial element in Laravel migrations. It is responsible for executing the predefined database schema. This section delved into the technical aspects of this technique, providing users with the necessary knowledge to utilize its capabilities effectively.
Essential Responsibilities of the Up() Method
Central hub for modifications: the up() method is a central processor unit. It executes the schema of the database modifications you’ve specified in the migration file. This includes a variety of actions, which include:
- New tables to create
- Add columns to tables
- The definition of constraints on columns (e.g. foreign keys, unique values)
- Establishing relations between tables
Utilizing Schema Builder Methods: The up() method employs the Schema Builder methods provided by Laravel. These methods give you extensive control over the various elements of your database’s schema and allow you to clearly specify the changes you want to make.
Public function to be held()
{
Schema::create(‘users’, function (Blueprint $table) {
$table->id();
$table->string(‘name’);
$table->string(’email’)->unique();
$table->timestamp(’email_verified_at’)->nullable();
$table->rememberToken();
$table->timestamps();
});
}
This up() method uses various Schema Builder methods, including creating strings, unique, and more. This allows you to build a “users” table with specific columns and restrictions. Each one converts your schema’s design into fundamental changes to your database.
Important Note: It is essential to be cautious when using an up() method since it will directly alter your database. Make sure you test and evaluate the migration in a non-production setting prior to making it available in your existing application.
Utilizing an up() technique and the Schema Builder strategies, you can perform well-defined schema changes to databases. These methods can help you build a solid base for data storage and capabilities.
Apply using the Down() Method (Optional)
Although not required, every Laravel migration may include a down() method. It is a safety cover if unexpected circumstances occur. Consider the function like an “undo” button. If needed, You can reverse the changes made by the “up() method.
The purpose of the method:()
Reversing Changes: A primary purpose for the down() method is to reverse any modifications made by the to the up() technique. This lets you reverse any changes you have made to your databases in the case of unforeseen situations. It can be used to address unpredictable behavior or security issues.
Testing and Debugging: The testing and debugging() method can also help conduct tests and debugging migrations. If you execute the down() method, you can restore your database to its initial state before rerunning the migration and making corrections.
Method of implementing the down() method:
Structure: Like the down() method, it employs Schema Builder methods to undo the modifications made in the down() technique. This includes:
- Dropping tables are created using the up() method.
- The removal of columns that were added to method up() method
public function down()
{
Schema::dropIfExists(‘users’);
}
In this case, the down() method employs the drop—ifExists method to remove users from the “users” table. The down() method reverses the changes made through the up() method.
Important note: While using the “down”() method can provide an important safety measure, it’s important to keep in mind that reversing database changes could lead to data loss. Be cautious when using this method, and ensure you have database backups before performing any changes.
Understanding the reason behind the implementation is the purpose and implementation() method. You can be empowered to design solid Laravel migrations. This will provide flexibility and recovery of the foundation for your application’s database schema.
Run the Migration
Let’s move on to the final step in translating your vision into reality: completing the migration to apply the specified changes to the actual database.
Command: Open your terminal, then navigate to your Laravel Project directory. Follow the steps below:
php artisan migrate
Useful: The command tells Laravel to handle all migrations in the queue that have yet to be executed. Each migration file is run sequentially, putting the changes you specify in your database.
Order of Execution: Laravel follows a specific sequence when performing the migration. The order of execution is determined based on the timestamp included in the filenames of every migration. This ensures that all migrations are performed in the correct sequence while maintaining the integrity of the database schema.
Database Connection: Prior to performing the migration, be sure the .env file contains the right credentials for your database. This lets Laravel connect with your database and run the migration commands correctly.
Check for Changes: Once you’ve completed the migration, it is recommended that you test the new changes within your database management system. Tools such as phpMyAdmin, MySQL, and Workbench can do this. This will ensure that the migration is completed correctly and that the modifications you want to make are applied to the appropriate tables.
When you run the migration procedure, you will be able to translate your database’s schema into measurable changes. This will provide a solid basis for the Laravel application to flourish.
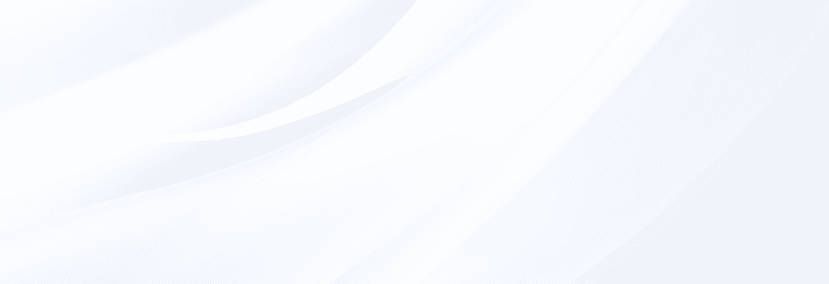
Transform Your Database with Ease! Get Started with Our Laravel Migration Services Today!
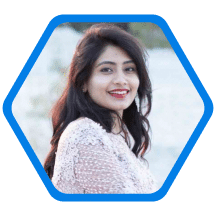
Pooja Upadhyay
Director Of People Operations & Client Relations
Advanced Laravel Migration Practices
Mastering the basics of Laravel migrations will give you an excellent foundation. Stepping into more advanced techniques can yield an abundance of advantages for developing your own process. This guide will outline five important techniques that can help you manage your schema database with greater performance, maintenance, and flexibility.
Schema Blueprints
It serves as a standardized component for building your database structure by defining reused components. This allows an organized code structure and eliminates redundancy during migrations. By using these predefined modules in your tables, you can build complicated databases.
// user_blueprint.php
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class UserBlueprint {
A public static method build(Blueprint $table) {public static function build(Blueprint $table)
$table->id();
$table->string(‘name’);
$table->string(’email’)->unique();
$table->timestamps();
}
}
Now, you can use this template with a variety of modifications:
// create_users_table.php
use UserBlueprint;
public function is up()
{
Schema::create(‘users’, function (Blueprint $table) {
UserBlueprint::build($table);
});
}
Custom Column Types
The Laravel core set of data types is a strong base, but sometimes you require more specific solutions. Custom column types enable users to expand this feature by creating data types tailored to a particular need. This allows you to organize and store complex data structures in your database.
Example:
use Illuminate\Database\Schema\Blueprint;
Public function to be held()
{
Schema::create(‘products’, function (Blueprint $table) {
$table->id();
$table->json(‘metadata’); // Custom JSON column type
$table->timestamps();
});
}
Key Regulations
Ensuring the accuracy and reliability of your information is essential. Key constraints, like primary keys and foreign keys, are the guardians of data integrity. These constraints establish essential relationships between tables, helping avoid invalid data and ensuring the overall condition of the database.
Example:
public function is up()
{
Schema::create(‘orders’, function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger(‘user_id’);
$table->foreign(‘user_id’)->references(‘id’)->on(‘users’);
$table->timestamps();
});
}
In this case, the user_id column within the “orders” table references the primary key (“id”) in the “users” table. This guarantees consistency and stops the creation of incorrect orders.
Batching Migrations
If you are faced with a variety of different migrations, consider batching them together to streamline your workflow. Laravel lets you group similar migrations into one file, streamlining the entire process and reducing the amount of work involved in individual migrations.
// create_feature_tables.php (batching multiple migrations)
public function is up()
{
Schema::create(‘table1’, function (Blueprint $table) {
// … table definition
});
Schema::create(‘table2’, function (Blueprint $table) {
// … table definition
});
/ … Additional table definitions
}
Seeding Factories
Laravel Seeding provides a convenient method to fill your database with accurate and consistent data samples, making it easier to test and develop. These factories function as generators of data. They allow you to generate various types of test data without the need for the manual process of creating your data.
Example:
// UserFactory.php (seeding factory)
$factory->define(App\Models\User::class, function (Faker $faker) {
Return
‘name’ => $faker->name,
’email’ => $faker->unique()->safeEmail,
‘password’ => Hash::make(‘password’),
];
});
By incorporating these advanced techniques and practices, you can enhance your Laravel transfer skills. In addition, this allows you to build and maintain a practical and durable base. If you are looking for a custom solution, it is possible to hire laravel developers. Their unique approaches and decades of experience guarantee that your Laravel applications will be successful and gain an edge over competitors.
How to Troubleshoot Common Laravel Migration Errors?
Even the most knowledgeable Laravel developers have moments of hiccups when transferring. This department provides you with the necessary knowledge to address five common issues with migrations and help you troubleshoot them effectively:
Syntax Errors
Warning: The migration fails to start, usually accompanied by confusing error messages.
Cause: The reason is incorrect syntax within the migration files, such as missing semicolons, mismatched parentheses, or grammatical errors in the method names.
Solution: Check your code for syntax mistakes. Make use of code editors with syntax highlighting tools or static analysis tools to spot possible issues. Make sure to double-check consistency and that the necessary statements are correctly ended.
Database Connection Issues
Warning: The failure of the migration and the message may signal a failure in connection or the inability to find the database.
Cause: The reason is the incorrect database credentials in your .env file. It could also be due to network connectivity issues or issues with the server hosting your database.
Solution: Check the authenticity of your database credentials within your .env file. Make sure your database server is up and accessible via your application. You can also use tools like PHP Artisan’s db:connect to test your database connection.
Duplicate Column Definitions
Warning: The migration is unsuccessful due to an error that indicates an identical column name in the table’s definition.
Cause: The reason is that you are trying to identify the same column in your transferring method.
Solution: Review your up method to ensure no column names are specified repeatedly. Verify that all columns have unique names in the table definition.
Missing or Incorrect Column Types
Warning: The migration fails, and the message could indicate a problem with an incorrect column type.
Reason: Do not mention the column type in the table definition or specify non-supported kinds of data for your column.
Solution: Use the Laravel documentation for an overview of the supported data types and ensure that every column has an appropriate type defined.
Insufficient Permissions
Warnings: The process is unsuccessful, with an error warning. This is a sign of problems with database permissions or with the user trying to run the.
Cause: The database user lacks ownership to perform the required operations (e.g., table creation, changing the existing table).
Solution: Grant the required rights to the person running the database migration. Ensure the user has the right privileges to create and edit tables in the database schema.
Recognising and fixing common mistakes will better equip you to overcome obstructions. It will also assist you in carrying out Laravel migrations with ease, clearing the way for a well-organized database foundation for your app.
Conclusion
Migrations with Laravel are a simple and effective method of controlling changes to your database’s schema over time, ensuring that your application is scalable and maintainable as it expands.
By following best practices when creating and managing Laravel changes, you will be able to develop efficient schema changes to your database that are compatible across multiple environments.
While they are not essential for creating a Laravel application, they are highly advised to ensure its stability, reliability, and maintenance.
Using Laravel migrations will save you time and effort when managing your database schema, allowing you to focus on enhancing the application’s core features and capabilities.
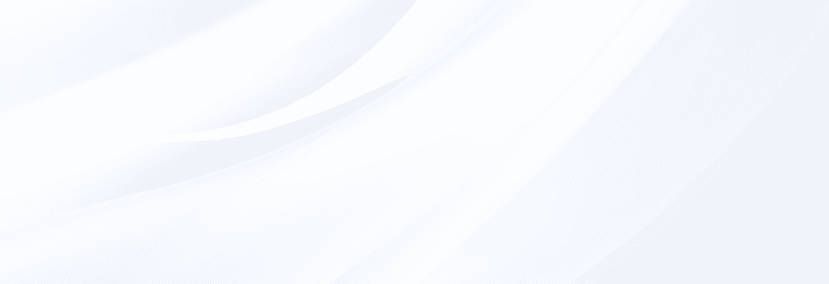
Don’t Let Database Issues Hold You Back! Hire Laravel Experts for Seamless Migrations!
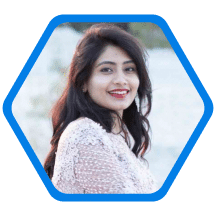
Pooja Upadhyay
Director Of People Operations & Client Relations